Working with Particle Wallet within Unity applications
Particle Wallet For Unity
Particle Wallet, within Unity applications, acts as a unified interface for both Web2 (via social login) and Web3 (via external wallets) accounts, working directly with Particle Connect. Particle Wallet on Unity provides games with a standard UI (yet customizable) UI for sending, swapping, and purchasing cryptocurrency natively within a corresponding account. Because of this, Particle Wallet is best described as wallet infrastructure, not a standalone wallet.
Details regarding the configuration and utilization of the Particle Wallet Unity SDK can be found below.
Repository
Before diving in, all of the Particle Network Unity SDKs (for Particle Auth, Particle Connect, and Particle Wallet) are publicly available and viewable through the official repository. Feel free to look at the underlying architecture and demos showcasing end-to-end implementation for an initial understanding of working with Particle Wallet on Unity.
Getting Started
Prerequisites
To start, your Unity project must meet several requirements to avoid compatibility issues. These requirements will be, in some capacity, dependent on the platform that you've decided to use. They are as follows:
- Unity 2021.3.35f1 or higher.
- If you're using iOS:
- Xcode 15.0 or higher.
- CocoaPods 1.14.3 or higher.
- iOS 14 or higher.
- If you're using Android:
- Minimum API level 23 or higher.
- Targets API level 33 or higher.
- Java SDK version 11.
Setting up the Particle dashboard
Once you've ensured your project meets the above prerequisites, you'll also need three key values from the Particle dashboard: your projectId
, clientKey
, and appId
. These directly connect your Unity project with the Particle dashboard, enabling customization, analytics, tracking, etc. To retrieve them, you'll need to head into the dashboard and create a new project, then a new application, as shown below:
- Sign up/log in to the Particle dashboard.
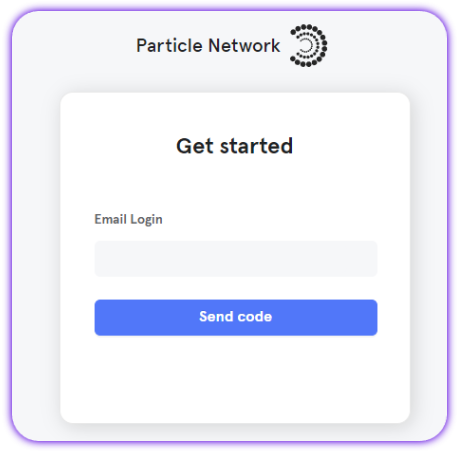
- Create a new project or enter an existing one.
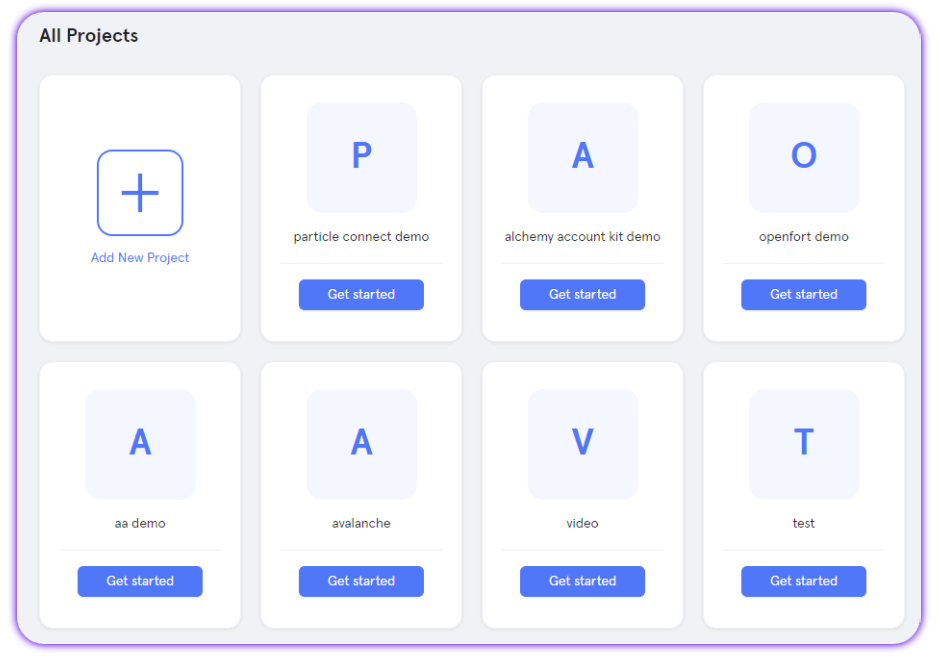
- Create a new application, or skip this step if you already have one.
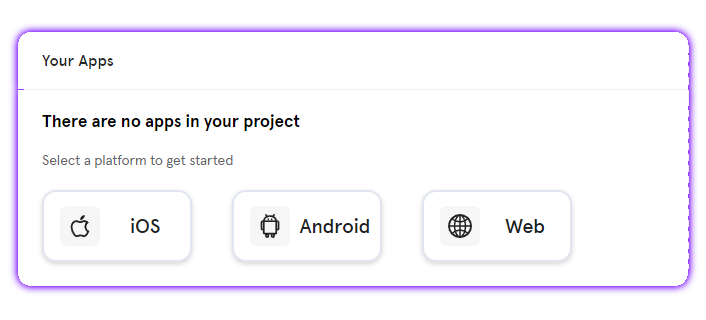
- Retrieve the project ID (
projectId
), the client key (clientKey
), and the application ID (appId
).
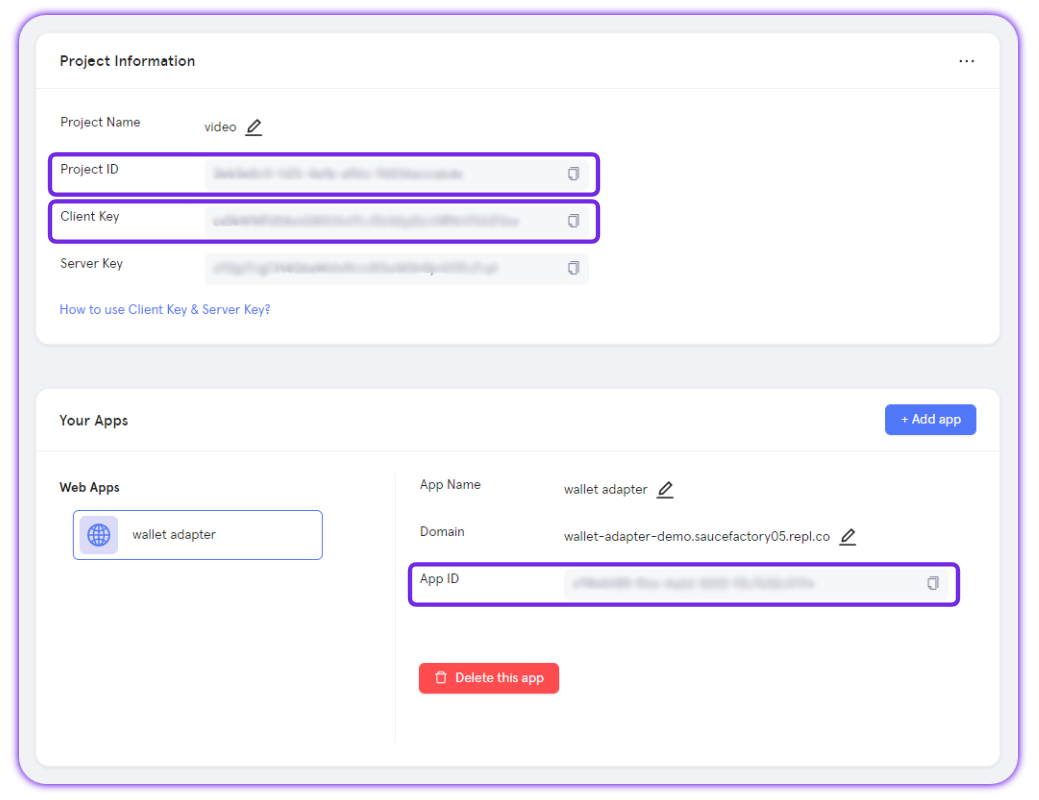
Configuration
With these values retrieved, you can move on to initial configuration and dependency management. This will vary in complexity depending on the platform in question. To begin, for both iOS and Android, you'll need to download and install the Particle Unity package.
Head over to the particle-unity
GitHub repository, and download the latest release (.unitypackage
), then import this into your project.
iOS configuration
If you're building a Unity game on iOS, you must follow a specific configuration process to ensure that Particle Wallet functions. The first step within this process is to set up a scheme URL within the Unity editor.
- Head into the iOS Player Settings menu (
Edit
->Project Settings
->Player Settings
->iOS
). - From here, select
Other
, then scroll down toConfiguration
. - Open the
Supported URL schemes
section, and within theElement 0
field, paste in yourprojectId
with a prefix ofpn
.
For example, if yourprojectId
(from the Particle dashboard) is something like63bfa427-cf5f-4742-9ff1-e8f5a1b9828f
, then the scheme URL, in this case, would bepn63bfa427-cf5f-4742-9ff1-e8f5a1b9828f
.
Select the specific services you intend to use.
Within
ParticleNetworkIOSBridge.cs
, you'll find a number of services included in the SDK:
ParticleNetworkBase
- required, universally.ParticleWalletGUI
- usage of the Particle Wallet UI, contains all services. RequiresSkeletonView
ParticleAuthService
- only if you're using Particle Auth directly.ParticleConnect
- if you're using Particle Connect directly.ParticleAuthCore
- only if you're using Particle Auth Core directly.
You'll also need to configure your Podfile if you haven't already. If you don't have a Podfile, go to the root of your project directory and run pod init
to generate one.
Open this Podfile, and insert the specific pods (services) you'd like to use within your project. In this case, ParticleWalletGUI
will generally suffice, but additional services can be added if needed.
Additionally, you'll need to paste in the below code snippet for installation handling:
pod 'ParticleWalletGUI'
// Also include:
pod 'SkeletonView', :git => 'https://github.com/SunZhiC/SkeletonView.git', :branch => 'main'
// Paste this
post_install do |installer|
installer.pods_project.targets.each do |target|
target.build_configurations.each do |config|
config.build_settings['BUILD_LIBRARY_FOR_DISTRIBUTION'] = 'YES'
end
end
end
With your Podfile set, you'll need to run pod install
and open your .xcworkspace
file, as shown below:
pod install --repo-update
open {your project}.xcworkspace
Finally, for iOS, you'll need to use the projectId
, clientKey
, and appId
previously retrieved. To do this, head into the root of your Xcode project and create a file, ParticleNetwork-Info.plist
. Within this file, paste the following text, then replace the placeholders:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<key>PROJECT_UUID</key>
<string>YOUR_PROJECT_UUID</string>
<key>PROJECT_CLIENT_KEY</key>
<string>YOUR_PROJECT_CLIENT_KEY</string>
<key>PROJECT_APP_UUID</key>
<string>YOUR_PROJECT_APP_UUID</string>
</dict>
</plist>
Android configuration
Alternatively, if you're building your Unity game for Android, you'll just need to configure two files before you're ready. The first of these two files can be found at Assets/Plugins/Android/launcherTemplate.gradle
within your project. Here, you'll need to ensure that you have the necessary dependencies included.
You'll need the following dependencies at a minimum:
network.particle:wallet-service
, required for Particle Wallet.network.particle:auth-service
, if you're planning on using Particle Auth directly.network.particle:connect
, if you're planning on using Particle Connect directly.network.particle:unity-bridge
, required universally.
dependencies {
implementation project(':unityLibrary')
implementation "network.particle:wallet-service:${sdkVersion}"
implementation "network.particle:unity-bridge:${sdkVersion}"
// Other dependencies
}
Finally, for Android, you'll need to place your projectId
, clientKey
, and appId
within gradleTemplate.properties
, found at Assets/Plugins/Android/gradleTemplate.properties
.
particle.network.project_client_key=Your Client Key
particle.network.project_id=Your Project ID
particle.network.app_id=Your App ID
With all of this set up, you can now drag the ParticleWalletGUI.prefab
file into your first scene.
Examples of Utilization
Initialization
With your Unity project configured and ready to go, you'll need to do one more thing before the full extent of the SDK is available: initialization. ParticleWalletGUI
will need to be initialized before Particle Wallet will work properly.
To do this, you'll need to call ParticleWalletGUI.ParticleWalletConnectInitialize
, passing in metadata
, which contains the following:
walletConnectProjectId
, your WalletConnect project ID retrieved from the WalletConnect dashboard.name
, the name of your project.icon
, a URL linking to the icon/logo of your project - ideally 512x512.url
, the URL of your project's website.description
, a short description of your project.- Optionally,
redirect
andverifyUrl
can also be added.
Additionally, if you're using ParticleNetwork
and ParticleConnectInteraction
for Particle Connect or Auth, you can initialize them in the same place and using the same metadata
.
var metadata = new DAppMetaData("WalletConnect project ID", "Particle Connect",
"https://connect.particle.network/icons/512.png",
"https://connect.particle.network",
"");
ParticleWalletGUI.ParticleWalletConnectInitialize(metaData);
// If applicable
ChainInfo chainInfo = new AvalancheChain(AvalancheChainId.Mainnet);
ParticleNetwork.Init(chainInfo);
ParticleConnectInteraction.Init(chainInfo, metadata);
Customization
Now that ParticleWalletGUI
is initialized and set to go, you can move onto configuring and customizing the wallet interface itself, choosing the specific options that are enabled and disabled within the wallet, the language used, and more. This can be done through ParticleWalletGUI.{configuration method}
. The specific methods available here include the following:
-
SetSupportChain
, sets the array of supported blockchain networks. It accepts an array of chain information, with the default being all chains. -
SetPayDisabled
, toggles the ability to perform buy transactions within the interface. It takes a Boolean,true
orfalse
, with no default value specified. -
SetShowTestNetwork
, controls the visibility of the test network. It accepts a Boolean value,true
orfalse
. -
SetShowManageWallet
, determines whether the manage wallet page is displayed. It takes a Boolean parameter,true
orfalse
. -
SetDisplayTokenAddresses
, specifies which token addresses are displayed, filtering out others. It accepts an array of token addresses to be displayed. -
SetDisplayNFTContractAddresses
, sets specific NFT contract addresses to be displayed, hiding others. It takes an array of NFT addresses. -
SetPriorityTokenAddresses
, prioritizes certain tokens to be shown at the top of the list. It accepts an array of token addresses. -
SetPriorityNFTContractAddresses
, sets certain NFTs to appear at the top of the list. It requires an array of NFT addresses. Similar toSetPriorityTokenAddresses
. -
SetSupportAddToken
, toggles the display of the add token button in the main wallet page. It takes a Boolean value,true
orfalse
. -
SetSupportWalletConnect
, controls support for wallet connect as a wallet option. It accepts a Boolean value,true
orfalse
. -
SetSupportDappBrowser
, determines whether the dApp browser should be shown on the wallet page. It takes a Boolean parameter,true
, orfalse
. -
SetShowLanguageSetting
, controls the visibility of the language setting button in the settings page. It accepts a Boolean parameter, eithertrue
orfalse
. -
SetShowAppearanceSetting
, toggles the display of the appearance setting button in the settings page. It takes a Boolean value,true
orfalse
.
E.g.:
ParticleWalletGUI.SetSupportChain(new []{chainInfo});
ParticleWalletGUI.EnablePay(false);
ParticleWalletGUI.SetShowTestNetwork(false);
ParticleWalletGUI.SetShowManageWallet(false);
ParticleNetwork.SetAppearance(UserInterfaceStyle.DARK);
ParticleWalletGUI.SetDisplayTokenAddresses(new []{"Your token address"});
ParticleWalletGUI.SetDisplayNFTContractAddresses(new []{"Your NFT address"});
ParticleWalletGUI.SetPriorityTokenAddresses(new []{"Your token address"});
ParticleWalletGUI.SetPriorityNFTContractAddresses(new []{"Your NFT address"});
ParticleWalletGUI.SetSupportAddToken(false);
ParticleWalletGUI.SetSupportWalletConnect(false)
ParticleWalletGUI.SetSupportDappBrowser(false)
ParticleNetwork.SetFiatCoin("HKD");
ParticleNetwork.SetLanguage(Language.KO);
ParticleWalletGUI.SetShowLanguageSetting(true);
ParticleWalletGUI.SetShowAppearanceSetting(true);
Open Wallet
Within ParticleWalletGUI
, you can also force specific pages of Particle Wallet to open, passing in specific preset configurations. In this case, you can programmatically open the main page of the wallet with NavigatorWallet
and pass in a binary (0
or 1
), indicating whether the main page should display ERC20/SPL tokens (0
), or NFTs (1
). E.g.:
int display = 0;
ParticleWalletGUI.NavigatorWallet(display);
Open Receive Token
Similarly, you can open a standalone page for receiving cryptocurrency. This'll include the user's address alongside a corresponding QR code. To do this, you'll need to call ParticleWalletGUI.NavigatorTokenReceive
. If you'd like, you can pass in a specific tokenAddress
to create a token-specific QR code, including its icon/logo at the center. E.g.:
ParticleWalletGUI.NavigatorTokenReceive();
ParticleWalletGUI.NavigatorTokenReceive(tokenAddress);
Open Send Token
To open the token sending page (ERC20/SPL/native), you'll can use ParticleWalletGUI.NavigatorTokenSend
, optionally passing in various parameters such as tokenAddress
, toAddress
, and amount
to predefine values within the interface. E.g.:
ParticleWalletGUI.NavigatorTokenSend(tokenAddress, toAddress, amount);
Open Transaction Records
You can also return a page displaying the transaction records of a connected wallet, ParticleWalletGUI.NavigatorTokenTransactionRecords
, which optionally takes one parameter, tokenAddress
, for filtering the results only to include transactions that involve a specified ERC20/721 token address. E.g.:
ParticleWalletGUI.NavigatorTokenTransactionRecords(tokenAddress);
Open NFT Send
Similar to the token sending page, you can also return an NFT sending page for moving (sending) a specific NFT to a different address. This can be done through ParticleWalletGUI.NavigatorNFTSend
, taking:
contractAddress
(the address of the NFT contract).tokenId
(the ID of the specific NFT belonging tocontractAddress
), can be left asnull
for Solana.receiveAddress
, the recipient, blank string by default.
E.g.:
ParticleWalletGUI.NavigatorNFTSend(contractAddress, tokenId, receiveAddress);
Open NFT Details
Particle Wallet also natively supports viewing specific NFTs (traits, description, image, etc.). Forcing this menu programmatically can happen through ParticleWalletGUI.NavigatorNFTDetails
, passing in the contractAddress
of the NFT and the specific tokenId
. For Solana, tokenId
can be left blank. E.g.:
ParticleWalletGUI.NavigatorNFTDetails(contractAddress, tokenId);
Open Buy Crypto
Particle Wallet also has a native onramp aggregator, allowing users to bring fiat on-chain through various onramp providers. Opening this programmatically can happen through ParticleWalletGUI.NavigatorBuyCrypto
, passing in several optional parameters to customize the values used within the onramp. Upon calling, this will throw a popup or total redirect over to a corresponding configuration of https://ramp.particle.network.
The specific parameters that can be used within ParticleWalletGUI.NavigatorBuyCrypto
are listed below:
Name | Description | Type | Required |
---|---|---|---|
network | [Solana, Ethereum, Binance Smart Chain, Polygon, Tron, Optimism, etc.] | string | False (True if Particle is not connected) |
fiatCoin | Fiat currency denomination. | string | False |
cryptoCoin | Cryptocurrency denomination. | string | False |
fiatAmt | The amount of fiat to be automatically filled in as the purchase volume. | number | False |
bool | Lock selection of fiat currency in the buy menu. | bool | False |
fixCryptoCoin | Lock selection of cryptocurrency in the buy menu. | bool | False |
fixFiatAmt | Lock selection of fiat amount in the buy menu. | bool | False |
walletAddress | The wallet address to receive the cryptocurrency. | string | False (True if Particle is not connected) |
BuyCryptoConfig config = new BuyCryptoConfig(TestAccount.EVM.PublicAddress,
OpenBuyNetwork.BinanceSmartChain, "BNB", "USD", 100);
ParticleWalletGUI.NavigatorBuyCrypto(config);
ParticleWalletGUI.NavigatorBuyCrypto();
Open Swap
Additionally, Particle Wallet has a built-in swap functionality (retrieves multiple quotes from different providers such as 1inch, iZUMi, etc., routing the swap through the best one) for Mainnets (Solana and EVM). Throwing this menu can be done through ParticleWalletGUI.NavigatorSwap
, which alone will open the default swap menu without values filled in, although you can pass in several optional parameters, including:
fromTokenAddress
, the token to swap from.toTokenAddress
, the token to swap to.fromTokenAmount
, the amount offromTokenAddress
to be automatically reflected within the UI.
E.g.:
ParticleWalletGUI.NavigatorSwap();
ParticleWalletGUI.NavigatorSwap(fromTokenAddress, toTokenAddress, fromTokenAmount);
Switch Wallet
Finally, before opening the UI, if you'd like to switch the WalletType
(walletType
in this case) reflected and used within the wallet itself, you can use ParticleWalletGUI.Instance.SwitchWallet
, passing in the specific walletType
(see Particle Connect iOS for more information) and targeted user address, publicAddress
. E.g.:
var walletType = WalletType.MetaMask;
var nativeResultData = await ParticleWalletGUI.Instance.SwitchWallet(walletType, publicAddress);
Master reference
For a direct, raw view into every method provided through ParticleWalletGUI
, below is a table containing every relevant one, alongside specific parameters and a short description. For methods listed that weren't covered in the above examples, live implementation often mimics the common structure covered throughout this document.
Class | Methods | Parameters (* indicates optional) |
---|---|---|
ParticleWalletGUI | NavigatorWallet | display |
ParticleWalletGUI | NavigatorTokenReceive | tokenAddress |
ParticleWalletGUI | NavigatorTokenSend | tokenAddress, toAddress, amount, modalStyle* |
ParticleWalletGUI | NavigatorTokenTransactionRecords | tokenAddress |
ParticleWalletGUI | NavigatorNFTSend | mint, tokenId, receiveAddress*, amount* |
ParticleWalletGUI | NavigatorNFTDetails | mint, tokenId |
ParticleWalletGUI | SetPayDisabled | disabled |
ParticleWalletGUI | GetPayDisabled | |
ParticleWalletGUI | SetSwapDisabled | disabled |
ParticleWalletGUI | GetSwapDisabled | |
ParticleWalletGUI | NavigatorBuyCrypto | config*, modalSyle* |
ParticleWalletGUI | NavigatorSwap | fromTokenAddress*, toTokenAddress*, amount*, modalStyle* |
ParticleWalletGUI | NavigatorLoginList | supportTypes |
ParticleWalletGUI | LoginListCallBack | json |
ParticleWalletGUI | SetShowTestNetwork | show |
ParticleWalletGUI | SetShowManageWallet | show |
ParticleWalletGUI | SetShowAppearanceSetting | show |
ParticleWalletGUI | SetShowLanguageSetting | show |
ParticleWalletGUI | SetShowSmartAccountSetting | show |
ParticleWalletGUI | SetSupportChain | chainInfos |
ParticleWalletGUI | SwitchWallet | walletType, publicAddress |
ParticleWalletGUI | SwitchWalletCallBack | json |
ParticleWalletGUI | SetSupportWalletConnect | enable |
ParticleWalletGUI | SetSupportDappBrowser | enable |
ParticleWalletGUI | ParticleWalletConnectInitialize | metaData |
ParticleWalletGUI | SetSupportAddToken | enable |
ParticleWalletGUI | SetDisplayTokenAddresses | tokenAddresses |
ParticleWalletGUI | SetDisplayNFTContractAddresses | nftContractAddresses |
ParticleWalletGUI | SetPriorityTokenAddresses | tokenAddresses |
ParticleWalletGUI | SetPriorityNFTContractAddresses | nftContractAddresses |
ParticleWalletGUI | LoadCustomUIJsonString | json |
ParticleWalletGUI | SetCustomLocalizable | localizables |
ParticleWalletGUI | SetCustomWalletName | name, icon |