Interacting with Particle Auth within applications made using Unreal Engine
Particle Auth for Unreal Engine
Extending beyond directly programmatic implementations, Particle Auth also features full support for Unreal Engine 5 through a no-code interface, enabling the integration of Particle's Wallet-as-a-Service in a familiar and straightforward environment when building games on Unreal Engine.
Getting Started
Getting started with the Particle Auth Unreal Engine SDK is simple, although it requires you to manually download and use the particle-unreal
GitHub repository to install the SDK.
To install:
- Open your project's root directly (you'll find your
.uproject
file here). - Create a new directory,
Plugins
, or skip this step ifPlugins
already exists. - Navigate to
Plugins/ParticleSDK
withinparticle-unreal
and copy it to yourPlugins
folder. - Open the Unreal Engine 5 editor, then head over to
Menu
, thenEdit
, and finallyPlugins
, in which you'll find an option to enableParticleSDK
.
Examples of utilization
Initialization
To begin, you'll need to create a config object with your projectId
, clientKey
, and appId
filled in. These are retrieved from the Particle dashboard through the following steps:
- Sign up/log in to the Particle dashboard.
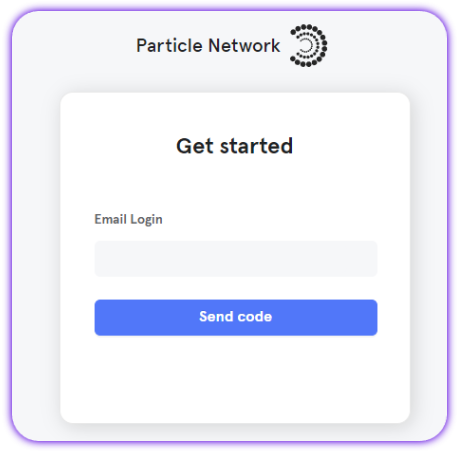
- Create a new project or enter an existing one.
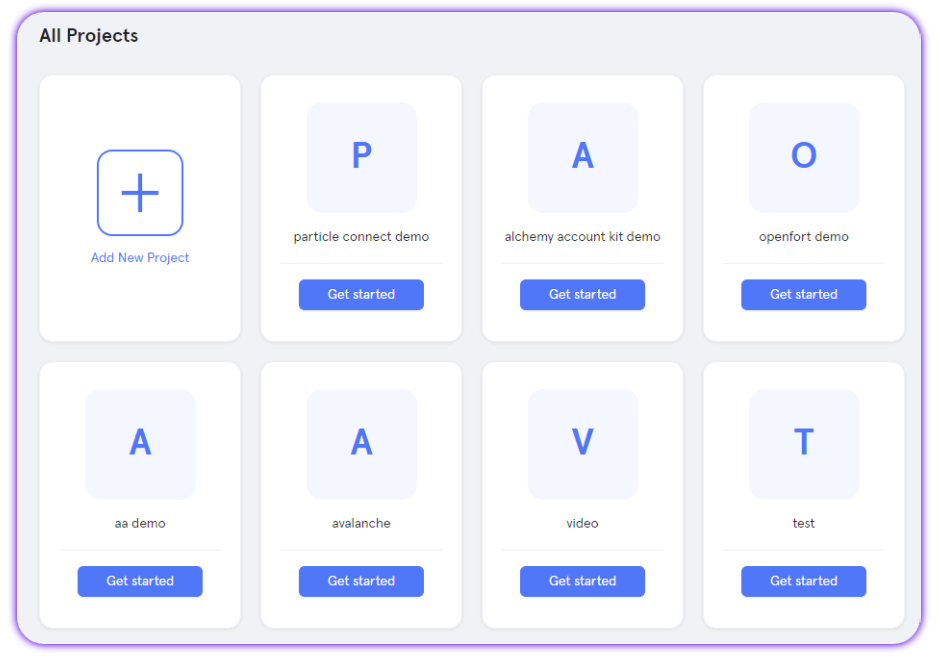
- Create a new web application, or skip this step if you already have one.
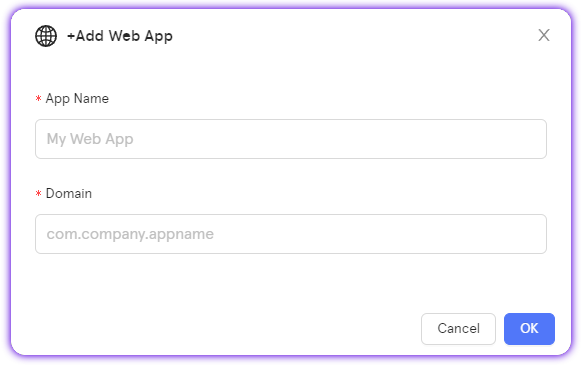
- Retrieve the project ID (
projectId
), the client key (clientKey
), and the application ID (appId
).
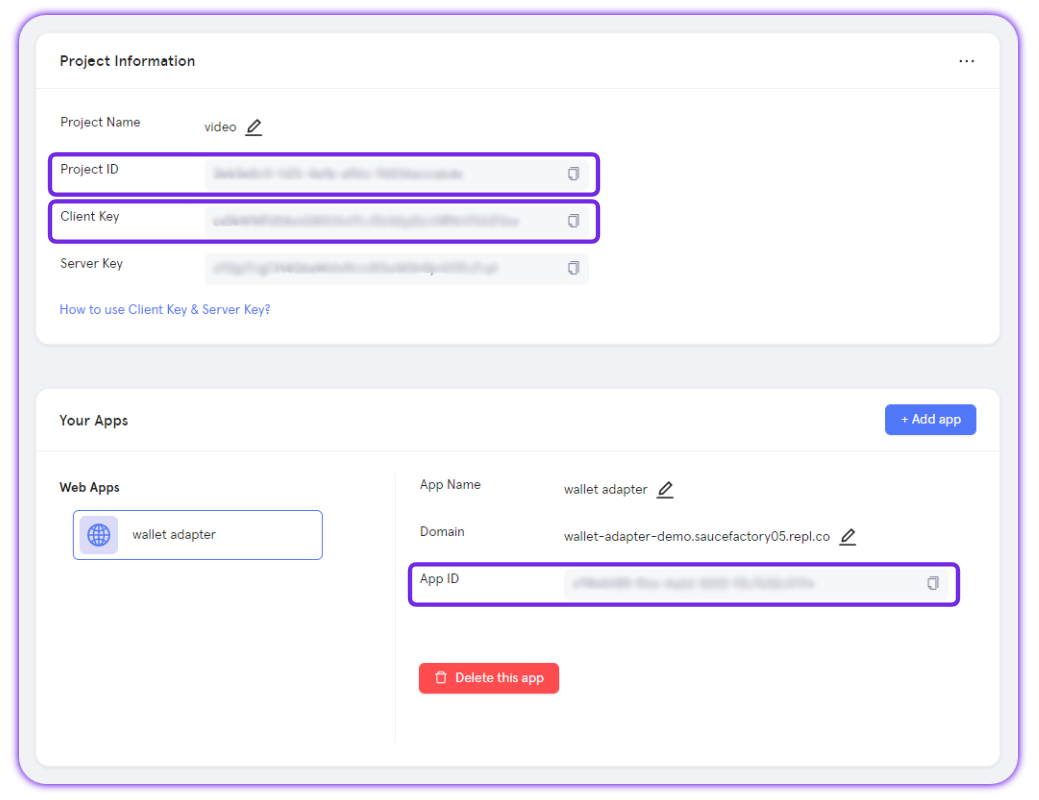
With these retrieved and set, you'll need to call the Init
function for formal initialization. The Init
function takes the following parameters:
Default Browser Widget
. Generally, this can be set toW_ExecuteWebBrowser
, although if you'd like to use a customWebBrowser
blueprint, you can use that instead (it must follow the structure ofW_ExecuteWebBrowser
).In Config
, which links back to the previously defined config object containingprojectId
,clientKey
, andappId
.In Theme
, an optional JSON string containing customization configuration. See Particle Auth Set Auth Theme for more information.In Language
, the language used within the modal, eitheren
,zh-cn
,zh-tw
,ja
, orko
.In Chain Name
&In Chain Id
; the primary chain to be used, either EVM or Solana. For more information on specific chainId and chainName configurations, see EVM Chains Structure.
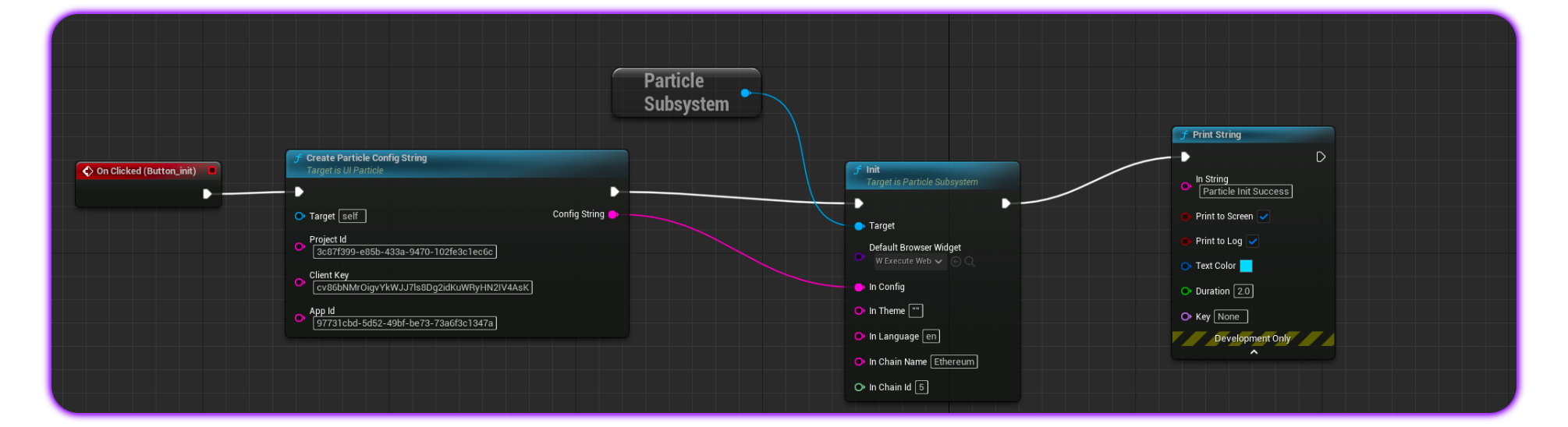
Login
Login
is the primary facilitator of onboarding and account creation/sign in within Particle Auth. On Unreal Engine, social logins can be accessed through the included Login
function, containing the following parameters:
Preferred Auth Type
, an optional field dictating the specific authentication type to be used. This should be either:phone
, for phone-based logins (sends a text message for verification and ties an account to a user's phone number).email
, for email-based login (sends a verification email and ties an account to a user email address).jwt
, for custom authentication (leveraging JWTs for external, custom authentication).
Account
, another optional field for passing expected values for an email address, phone number, or JWT.
Upon successful login, a JSON string containing detailed user info (email or phone, addresses, UUID, token, etc.) will be returned as event data.
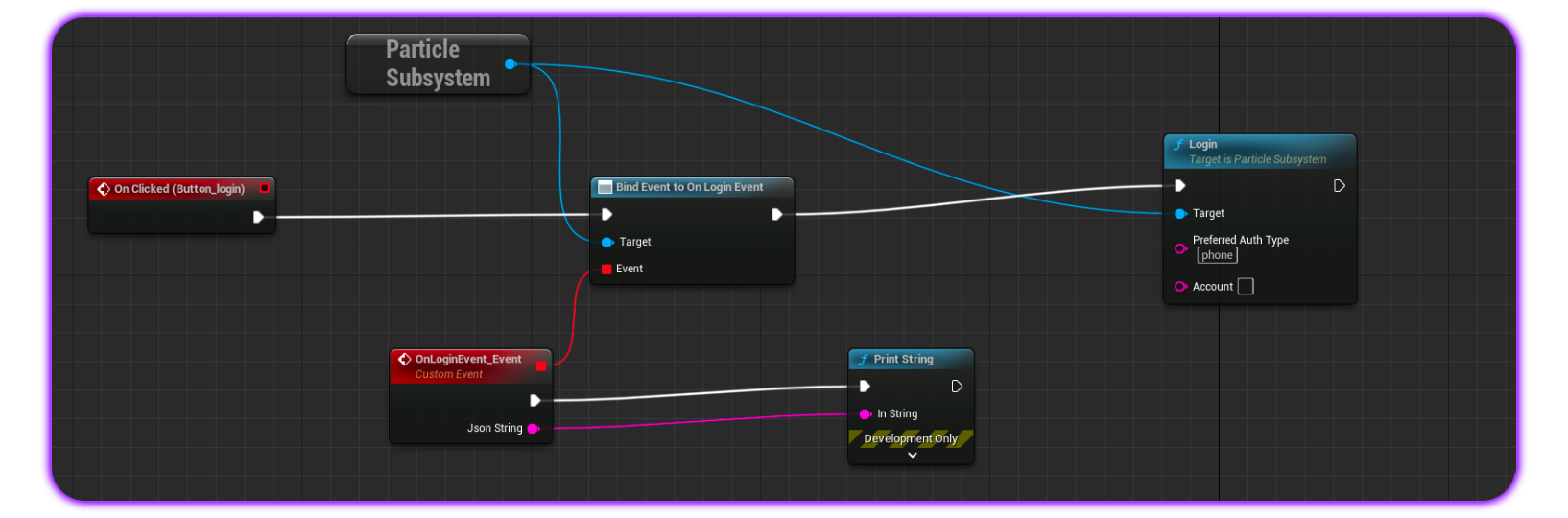
Sign Message
For the prompting of a standard message signature (general strings, not typed data), you can use the SignMessage
blueprint, which displays a signature request for the end-user to confirm. SignMessage
contains the following parameters:
Message
, the message to be signed by the user. For EVM chains, this can be passed as a standard UTF-8 string, although for Solana, this should be a base58 string.
Upon successful confirmation, the signature will be returned in event data via OnSignMessageEvent
.
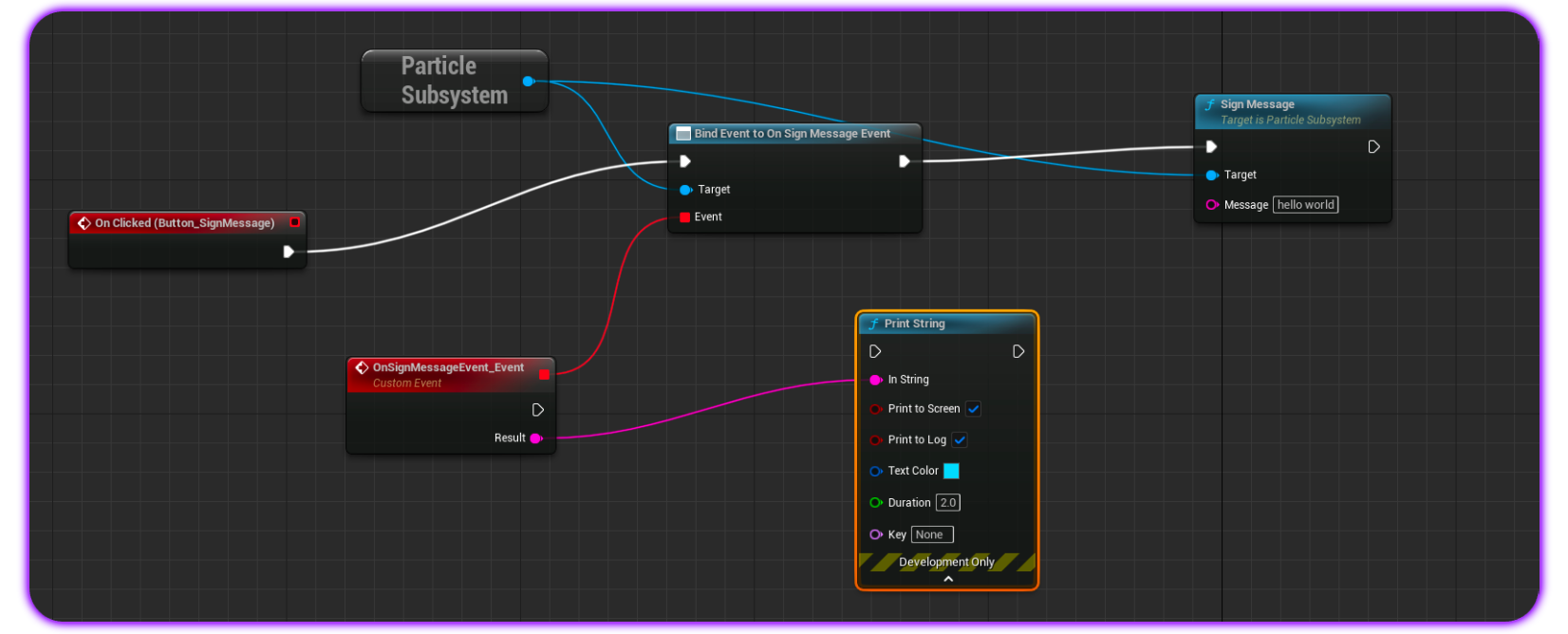
Sign and Send Transaction
SignAndSendTransaction
acts as the foundational method for sending transactions on EVM and Solana. Upon calling, a transaction will be thrown and displayed to an end-user, in which they can either confirm a signature and push the transaction to the network or reject it and cancel the operation. The SignAndSendTransaction
blueprint takes the following parameters:
Transaction
, this should be a string (standard UTF-8 for EVM, base58 for Solana) representing a transaction object/structure to be sent (can be a stringified object).
Additionally, you can use MakeEvmTransaction
, a helper method that can be attached to Transaction
on SignAndSendTransaction
for no-code generation of an EVM transaction.
Upon a successful signature, OnSignAndSendTransactionEvent
will include event data with the signature present.
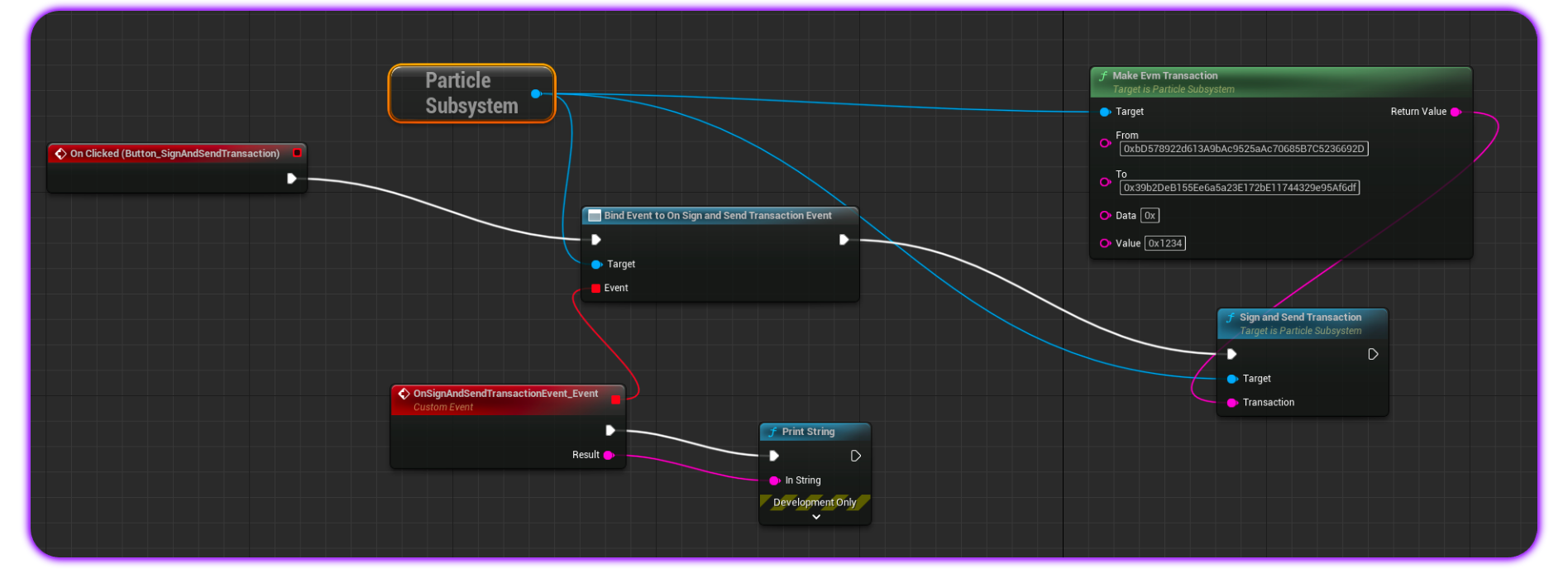
Sign Typed Data
On EVM chains, as an alternative to SignMessage
, you can use SignTypedData
to prompt a signature of structured (typed) data. The SignTypedData
blueprint takes the following parameters:
Message
, the message (or data in this case) to be prompted for signature. This should be a standard JSON string containing a data structure.Version
, Particle Auth supports all three versions ofeth_signTypedData
;v1
,v3
, andv4
. By default,v4
is used.
Upon confirmation, OnSignTypedDataEvent
will return the signature in question.
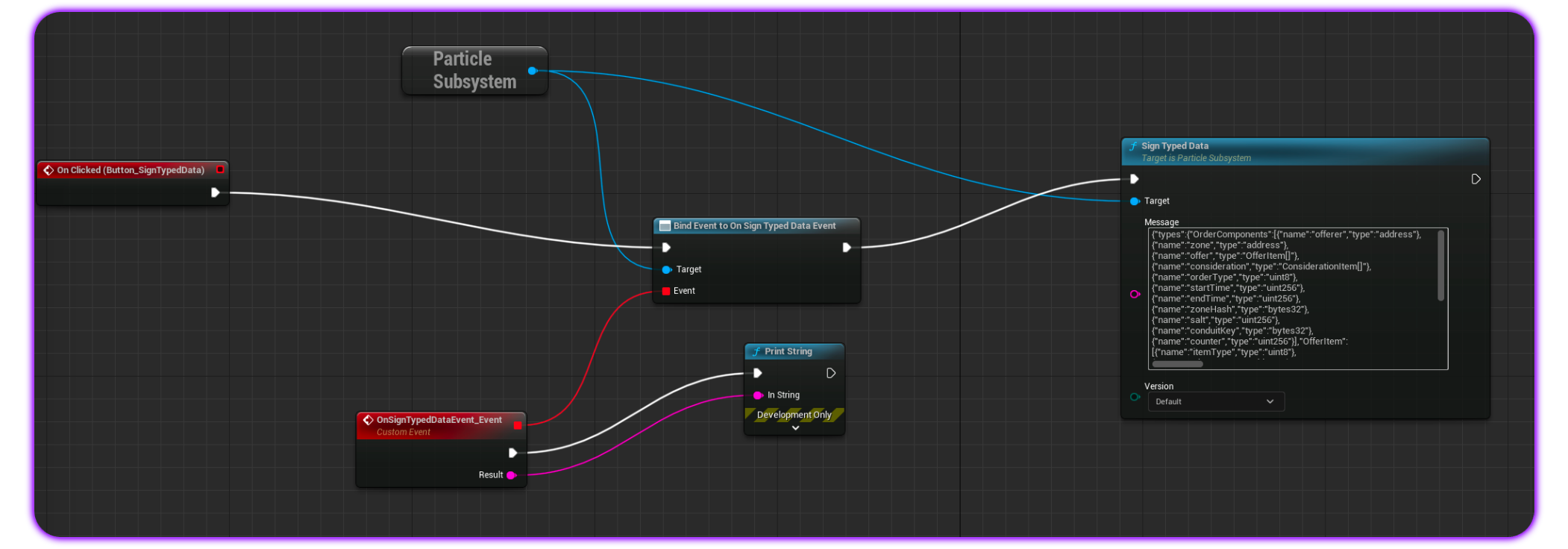
Sign Transaction
SignTransaction
is a Solana-specific method for signing (and thus returning a signature for) a given transaction without pushing it to the network. The SignTransaction
blueprint takes the following parameter:
Transaction
, this should be a base58 string representing a valid transaction structure to be prompted for signature.
Upon a successful signature, OnSignTransactionEvent
will return the completed signature in question.
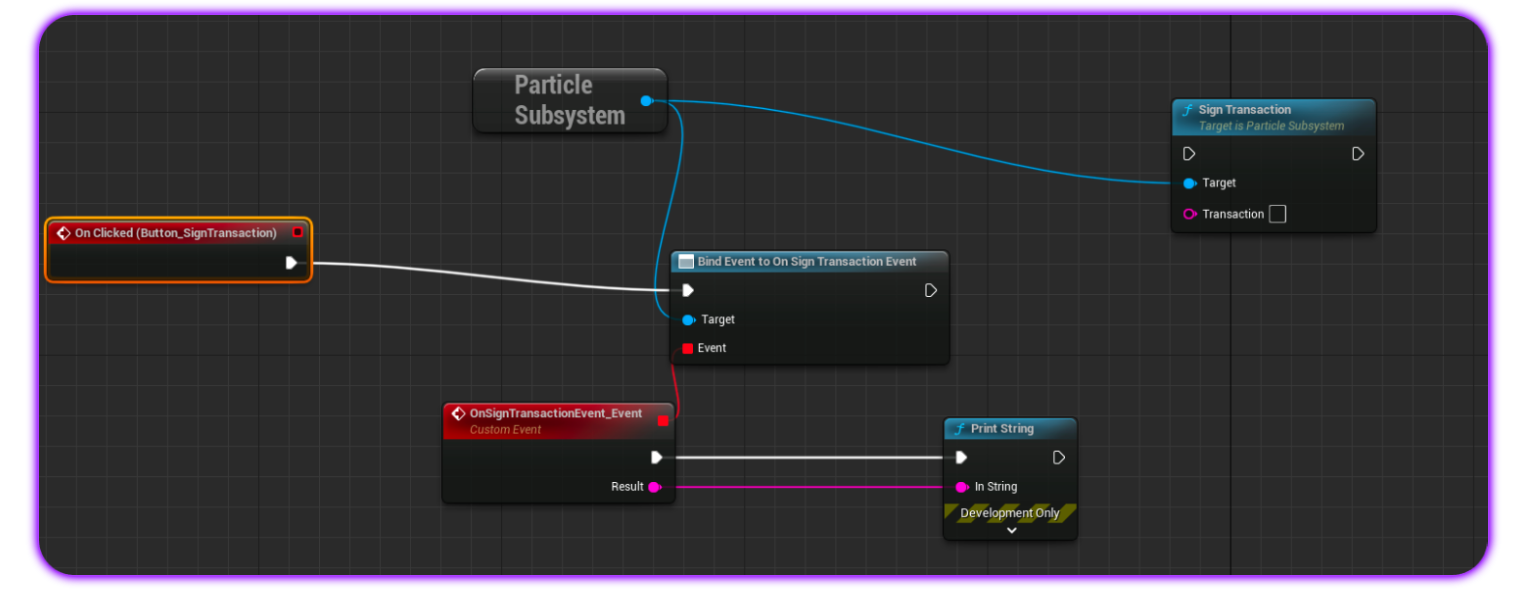
Sign All Transactions
The plural of the former, SignAllTransactions
is another Solana-specific method for collectively signing transactions without pushing them to the network. The SignAllTransactions
blueprint takes the following parameter:
Transactions
, this should be an array of base58 strings, each representing a valid transaction to be included and prompted for signature.
After this function concludes and signatures are generated, OnSignAllTransactionsEvent
will return them.
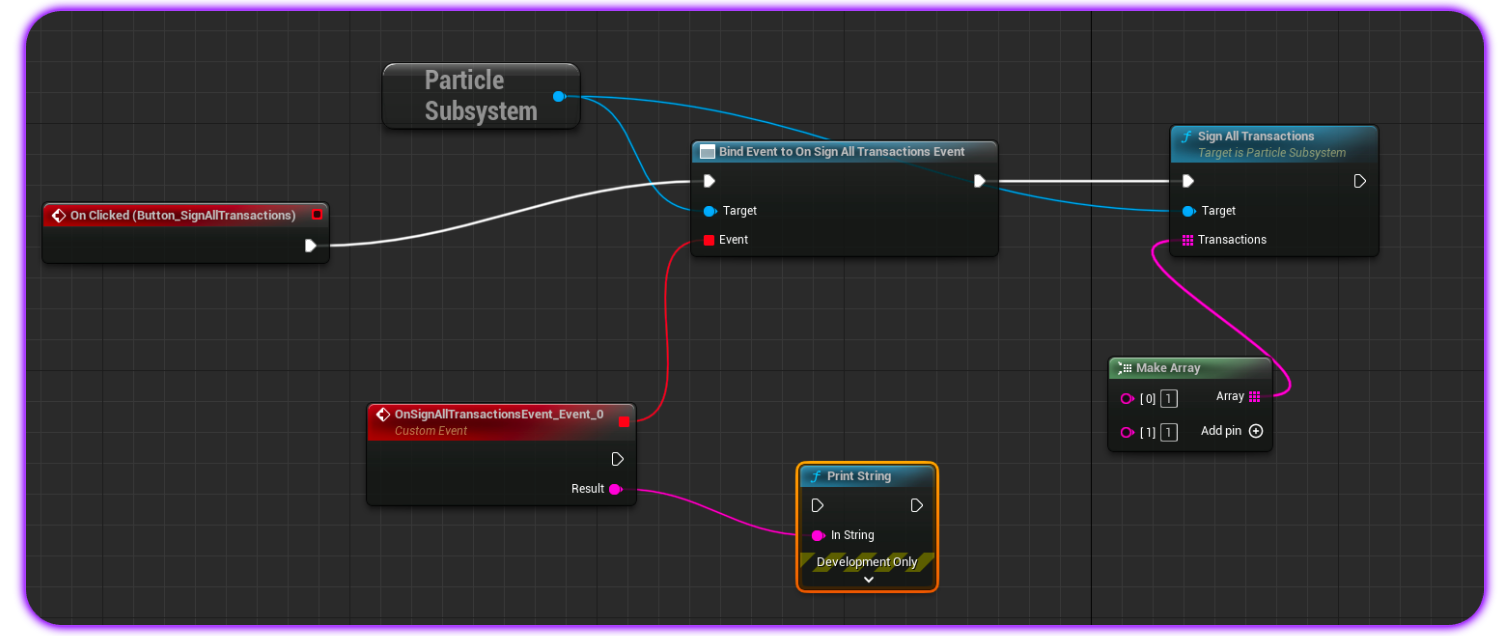