Interacting with Particle Auth within games built on Unity using C#
Particle Auth for Unity on Windows/macOS
The Particle Auth Unity SDK acts as the primary mechanism for integrating Particle's Wallet-as-a-Service within games built on Unity. Specifically, this SDK facilitates configuration, integration, and interaction. This means that, for most applications, the Particle Auth's Unity SDK can be the sole library backing end-to-end utilization of Particle Network facilitated by users' email, phone, or custom JWT authentication.
The structure of this SDK follows the Web (JavaScript/TypeScript) page closely, albeit with several deviations, as described below.
Due to limitations with Webview, Particle Auth for Unity on Windows & macOS does not include most built-in social logins
Options include email, phone, and custom JWT authentication (indicated by 'email', 'phone', or 'jwt').
Getting Started
To begin utilizing the Particle Auth Unity SDK on Windows & macOS, you'll need to meet a few prerequisites, specifically a minimum version of Unity along with a Unity package to handle authentication popups.
Prerequisites
-
Unity 2020.3.26f1 or later.
-
Requires one of the following packages:
-
Recommended: 3D WebView for Windows and macOS (Web Browser), version 4.3.3.
-
Other web browser packages will also work here if necessary.
-
Quickstart
Before diving into the specifics of SDK initialization and utilization, the best way to get started and explore a live implementation of the Unity SDK is through the Windows/macOS demo included within the GitHub repository. This demo will include the initialization of the SDK and, as previously detailed, a Webview-based authentication menu for MPC-TSS login, enabling interaction with the resulting wallet.
Quickstart demo installation
To get started and begin testing the included demo, you'll need to first download the Windows/macOS demo here. Assuming you've previously imported the SDK, also found in the linked repository, you can initialize the demo. To do this:
- Open the Windows/macOS Auth demo scene within the Unity editor.
- Click the
Particle Init
button to initialize the SDK.
- Finally, click
Login
to initiate an account instance and enable the sample functions.
Initialize
After exploring an implementation of the SDK through the demo, let's understand and dive into configuration alongside some of the structural deviations from the Web SDK.
To initialize the Particle Auth SDK, go ahead and create a GameObject
and attach the ParticleSystem
script, then attach a CanvasWebViewPrefab
to ParticleSystem
script.
Before utilization, you'll need to configure ParticleSystem
(adjacent to the Web SDK's ParticleNetwork
). This configuration can be executed by calling the Init
method on ParticleSystem.Instance
with parameters as follows:
-
config
: JSON string with universal configurations, such as the requiredprojectId
,clientKey
, andappId
. -
theme
: Optional JSON string for dictating specific customization. -
language
: String indicating the language to be used within the UI, either 'en-US', 'zh-CN', 'zh-TW', 'ja-JP', or 'ko-KR'. -
chainName
andchainId
: A string and integer indicating the primary chain to be used.
var config = new ParticleConfig();
config.ProjectId = "7fa3e77f-9d07-4417-8e45-01560fef0eab";
config.ClientKey = "cRX26iCgKipWu6scQi6R8qWaP903EF3YsL3bxIym";
config.AppId = "4ca94b0e-74b9-4a5b-aad3-72ee1ed84793";
// Retrieved from https://dashboard.particle.network
var theme = new ParticleTheme();
theme.UiMode = "dark";
theme.DisplayWallet = true;
theme.DisplayCloseButton = true;
string language = "en";
string chainName = "Ethereum";
long chainId = 5;
ParticleSystem.Instance.Init(config.ToString(), theme.ToString(), language, chainName, chainId);
Among these parameters, the config
JSON string containing projectId
, clientKey
, and appId
is the most important. It directly connects your instance of Particle's Wallet-as-a-Service with your configurations in the Particle dashboard. You can retrieve the values for these three parameters through the following:
- Sign up/log in to the Particle dashboard.
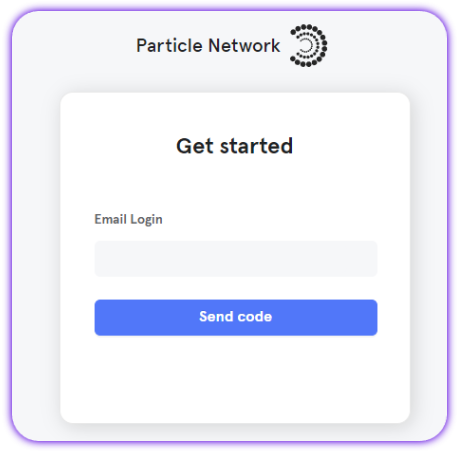
- Create a new project or enter an existing project.
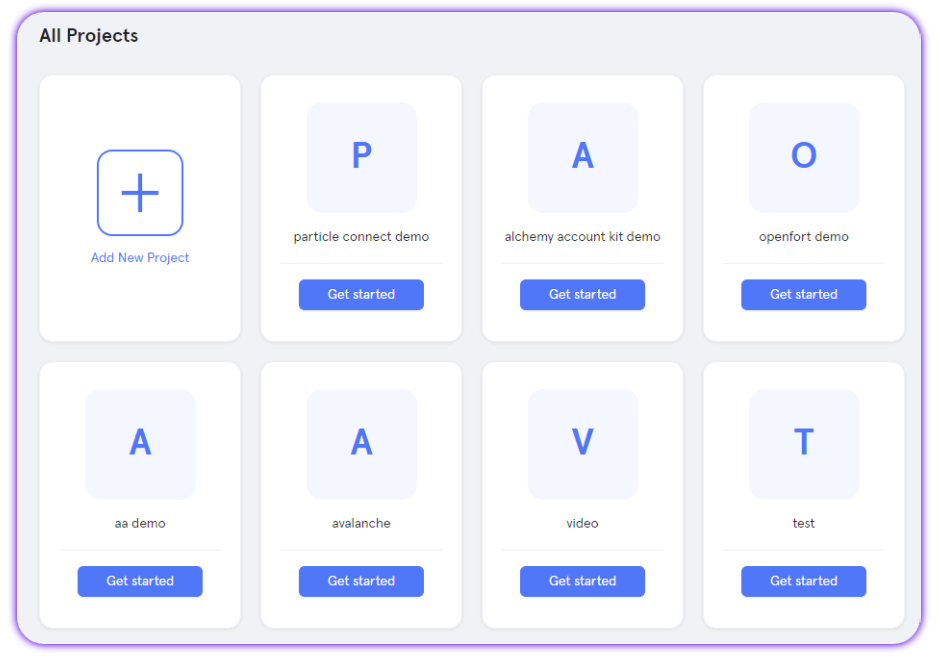
- Create a new web application, or skip this step if you already have one.
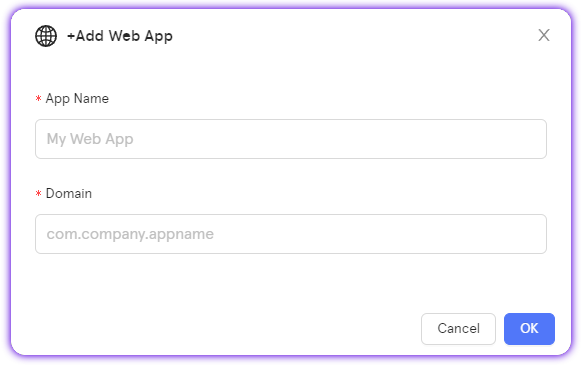
- Retrieve the project ID (
projectId
), the client key (clientKey
), and the application ID (appId
).
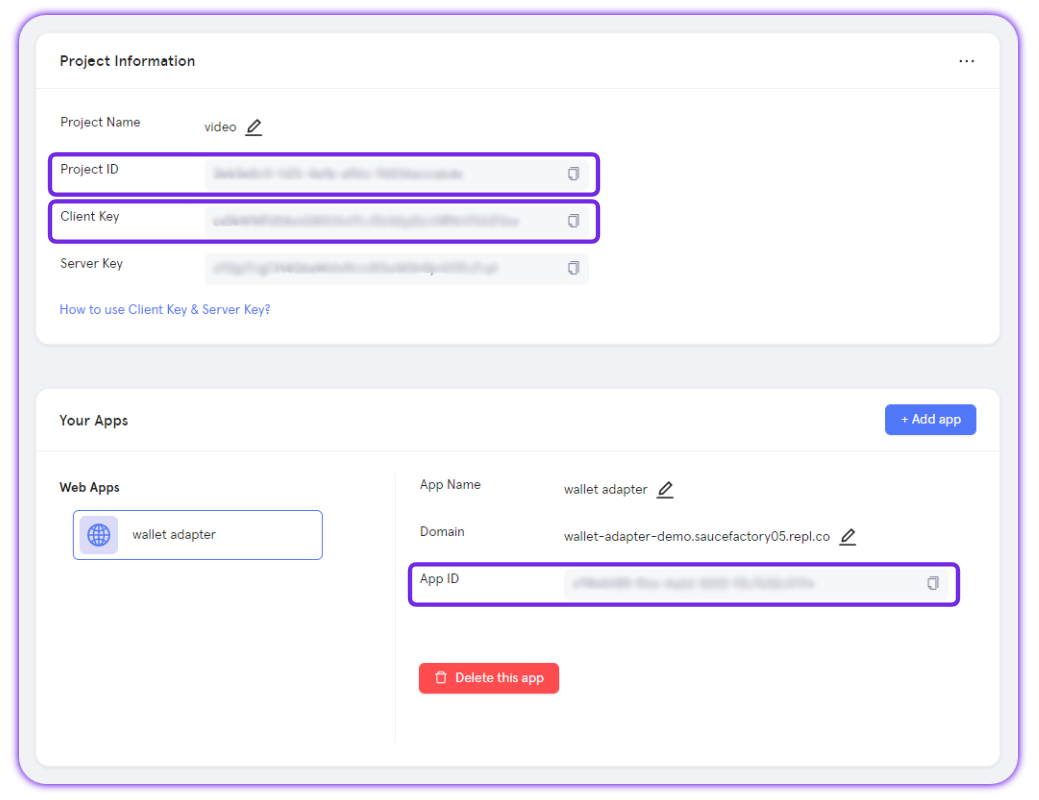
Examples of utilization
Login
ParticleSystem.Instance.Login
displays the login popup, set to either 'email', 'phone', or custom JWT authentication with 'jwt'. This popup acts as the primary mechanism for facilitating account creation.
// PreferredAuthType: email, phone, or jwt
// Account: Required when using jwt
var loginResult = await ParticleSystem.Instance.Login(PreferredAuthType.email, "");
Debug.Log($"Login result {loginResult}"); // Contains user information
SignMessage
ParticleSystem.Instance.SignMessage
enables the signing of a basic string. For EVM chains, this can be passed directly within SignMessage
, as shown below. For Solana, you'll need to convert this string to base58 before SignMessage
will function properly. The confirmation popup will display this string, base58 or otherwise, in UTF-8.
var signMessageResult = await ParticleSystem.Instance.SignMessage("GM, Particle!");
Debug.Log($"SignMessage result {signMessageResult}");
SignAndSendTransaction
ParticleSystem.Instance.SignAndSendTransaction
takes in a constructed transaction object returned from ParticleSystem.Instance.MakeEvmTransaction
(for EVM chains), then requests a confirmation (signature) from the user. Once granted, it sends the transaction.
var transaction = ParticleSystem.Instance.MakeEvmTransaction("0x16380a03f21e5a5e339c15ba8ebe581d194e0db3", "0xA719d8C4C94C1a877289083150f8AB96AD0C6aa1", "0x", "0x123123");
var signMessageResult = await ParticleSystem.Instance.SignAndSendTransaction(transaction);
Debug.Log($"SignAndSendTransaction result {signMessageResult}");
SignTypedData
ParticleSystem.Instance.SignTypedData
facilitates the signature of structured data; by default, this uses eth_signTypedData_v4
. For more information on signTypedData
, see the Web (JavaScript/TypeScript) page.
string typedDataV4 = "";
var signMessageResult = await ParticleSystem.Instance.SignTypedData(typedDataV4, SignTypedDataVersion.Default);
Debug.Log($"SignTypedData result {signMessageResult}");
SignTransaction
ParticleSystem.Instance.SignTransaction
is a Solana-specific method for requesting a signature for a given transaction. This will sign (but not send) a transaction.
string transaction = "";
var signMessageResult = await ParticleSystem.Instance.SignTransaction(transaction);
Debug.Log($"SignTransaction result {signMessageResult}");
SignAllTransactions
ParticleSystem.Instance.SignAllTransactions
is the plural of the aforementioned; this is also Solana-specific.
List<string> transactions = new List<string> { "" }; // base58 strings
var signMessageResult = await ParticleSystem.Instance.SignAllTransactions(transactions);
Debug.Log($"SignAllTransactions result {signMessageResult}");
Master reference
For a direct, raw view into every method provided through ParticleSystem
, below is a table containing every relevant method alongside specific parameters and a short description. For methods listed that weren't covered in the above examples, live implementation often mimics the common structure covered throughout this document.
Class | Methods | Parameters |
---|---|---|
ParticleSystem | Init | config, theme, language, chainName, chainId |
ParticleSystem | Login | preferredAuthType, account |
ParticleSystem | SignMessage | message |
ParticleSystem | SignAndSendTransaction | transaction |
ParticleSystem | SignTypedData | message, version |
ParticleSystem | SignTransaction | transaction |
ParticleSystem | SignAllTransactions | transactions |
ParticleSystem | MakeEvmTransaction | from, to, data, value |
ParticleSystem | UpdateChainId | chainId |
ParticleSystem | UpdateChainName | chainName |