Mobile (Android/iOS) Quickstart
Implementing Particle's Wallet-as-a-Service within Mobile Applications
(est. time: less than 5 minutes)
Most of the 32 SDKs available within Particle Network's Wallet-as-a-Service belong to mobile platforms. Particle's Wallet-as-a-Service has SDKs across Android, iOS, Unity, Flutter, and React Native. Across these various SDKs, the implementation flow of the associated services (Particle Auth, Particle Auth Core, Particle Connect, and the Particle AA SDK) varies significantly based on the specific platform, although there are several common denominators; these will be covered within this document.
For a full rundown on each service and its associated mobile SDKs (with detailed tutorials on installation, configuration, and utilization), see Introduction to API & SDK overview.
Installing WaaS-related libraries
Between Particle Auth, Particle Auth Core, Particle Connect, Particle Wallet, and the Particle AA SDK, there are a variety of potential SDKs you could use to implement these services within your application. The specifics of which ones you choose will depend mainly on the platform you intend to build your application on.
A rundown of the various core platform-specific mobile SDKs for each service can be found below:
Product | SDKs | Description |
---|---|---|
Particle Auth | network.particle:auth-service (Android), ParticleAuthService (iOS), particle_auth (Flutter), particle-unity (Unity), @particle-network/rn-auth (React Native), particle-cocos (Cocos) | The various platform-specific mobile Particle Auth SDKs act as an all-in-one mechanism for onboarding users (initiating social login) and interacting with accounts created through Particle's Wallet-as-a-Service. |
Particle Auth Core | network.particle:auth-core , network.particle:mpc-core (Android), ParticleAuthCore , ParticleMPCCore (iOS), particle_auth_core (Flutter), rn-auth-core , rn-auth (React Native), ParticleAuthCore (Unity) | As an alternative to Particle Auth, Particle Auth Core handles social logins and account interaction, although with a greater degree of customizability and a slightly altered implementation flow. |
Particle Connect | particle.network:connect (Android), ParticleConnect (iOS), particle_connect (Flutter), @particle-network/rn-connect (React Native) | The Particle Connect mobile SDKs enable unified connection between Web2-based social logins and external wallets through an in-house connection kit. |
Particle Wallet | network.particle:wallet-service (Android), ParticleWalletAPI , ParticleWalletGUI (iOS), particle_wallet (Flutter), @particle-network/rn-wallet (React Native) | Particle Wallet and its associated mobile SDKs act as wallet infrastructure for accounts generated by social login with Particle Auth alongside external wallets connected through Particle Connect. |
Particle AA SDK | network.particle:aa-service (Android), ParticleAA (iOS), particle_aa (Flutter), @particle-network/rn-aa (React Native), ParticleAA (Unity) | The Particle Network AA SDK enables end-to-end utilization of account abstraction natively within Particle Network's Wallet-as-a-Service, handling smart accounts, the construction and sending of UserOperations, etc. |
Configuring Particle Auth
As mentioned, using these SDKs will vary significantly, although there are some common structures to keep in mind as you implement them within your application. The first and most universal of them is the requirement of the projectId
, clientKey
, and appId
values. Each SDK, regardless of platform, will require these three values for authentication and connection between your application and the Particle dashboard.
To find these values and use them within your project, follow these steps:
- Sign up/log in to the Particle dashboard.
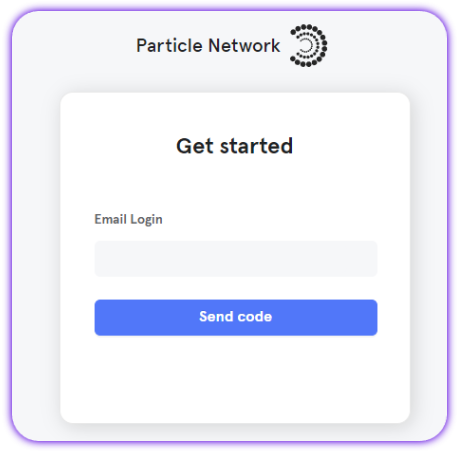
- Create a new project or enter an existing project.
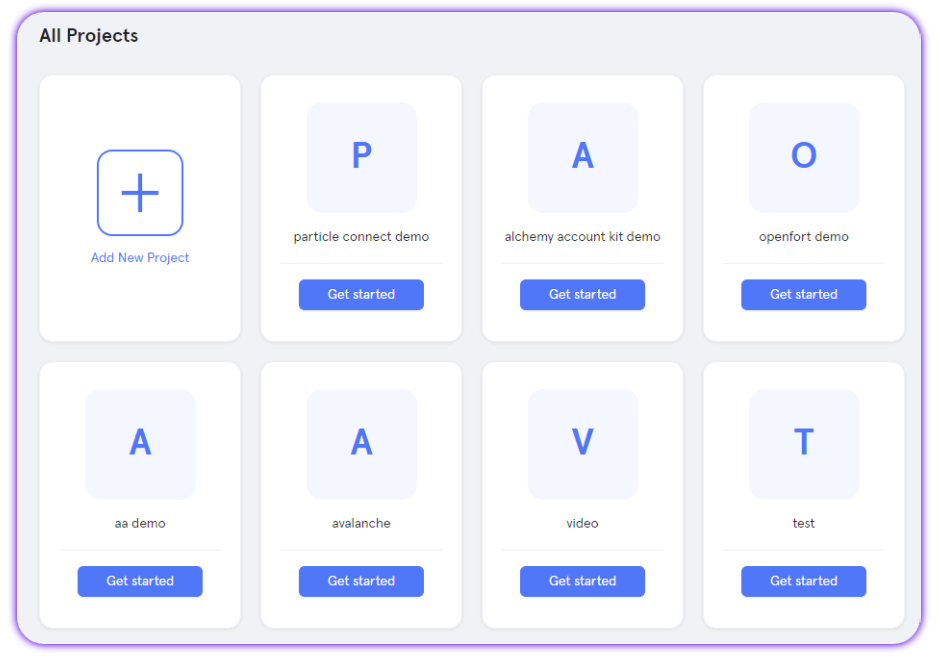
- Create a new application, or skip this step if you already have one.
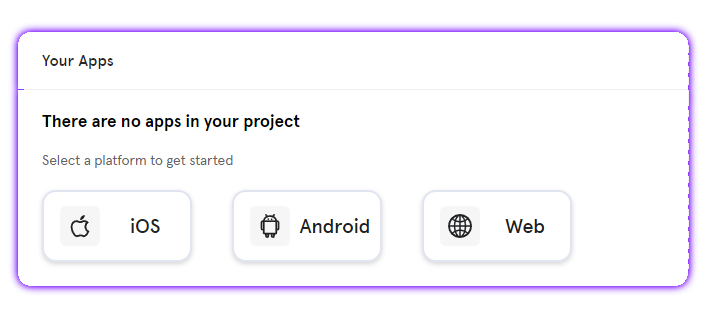
- Retrieve the project ID (
projectId
), the client key (clientKey
), and the application ID (appId
).
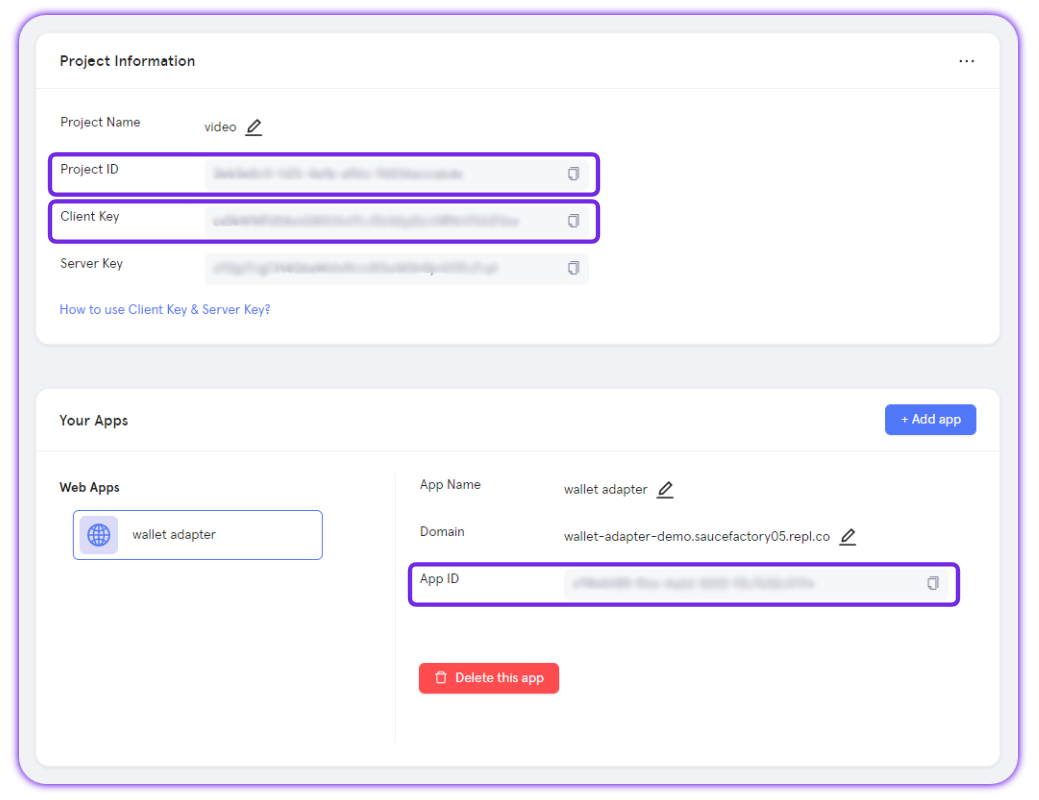
For more information on the Particle dashboard, take a look at this video.
Now that you have your projectId
, clientKey
, and appId
, you can move on to configuring your SDK of choice. The configuration will look somewhat similar between each service, although, of course, with changes in structure and syntax between platforms. To understand where you need to enter your projectId
, clientKey
, and appId
, head over to Introduction to Particle Auth and find the guide for your platform of choice.
Once you've configured the SDK in the backend, initialization will look like one of the following snippets, depending on your platform:
// Android
ParticleNetwork.init(this, Env.DEV, EthereumChain(EthereumChainId.Mainnet))
// iOS
let config = ParticleNetworkConfiguration(chainInfo: chainInfo, devEnv: devEnv)
ParticleNetwork.initialize(config: config)
// Flutter
ParticleAuth.init(Ethereum.mainnet(), env);
// Unity
ParticleNetwork.Init(ChainInfo.Ethereum);
// React Native & Cocos
particleAuth.init(chainInfo, env);
Facilitating login
Like the configuration and initialization mechanism, the specific syntax and setup required for initiating a social login within your mobile application will deviate based on the platform. However, generally, this can be done by calling the login
method available within the master object used prior for initialization (ParticleNetwork
, ParticleAuth
, particleAuth
), and passing in common parameters, as is shown below:
// Android
ParticleNetwork.login(
loginType = LoginType.PHONE,
account = "", // Optional
supportAuthTypeValues = SupportAuthType.FACEBOOK.value, // Optional
loginFormMode = false, // Optional
prompt = null, // Optional
loginCallback = object : WebServiceCallback<LoginOutput> {
override fun success(output: LoginOutput) {
// Handle success
}
override fun failure(errMsg: WebServiceError) {
// Handle failure
}
})
// iOS
ParticleAuthService.login(type: .email)
.subscribe { [weak self] result in
guard let self = self else { return }
switch result {
case .failure(let error):
// Handle failure
case .success(let userinfo):
// Handle success - returns userinfo
}
}.disposed(by: bag)
// Flutter
ParticleAuth.login(
LoginType.phone,
"",
supportAuthType,
socialLoginPrompt,
authorization
);
// Unity
await ParticleAuthService.Instance.Login(LoginType.EMAIL,
null,
SupportAuthType.ALL,
SocialLoginPrompt.SelectAccount,
authorization
);
// React Native & Cocos
await particleAuth.login(type, '', supportAuthType);
The complete mobile SDK reference is available at SDKs (Mobile)
Updated 3 months ago